思考并回答以下问题:
middleware
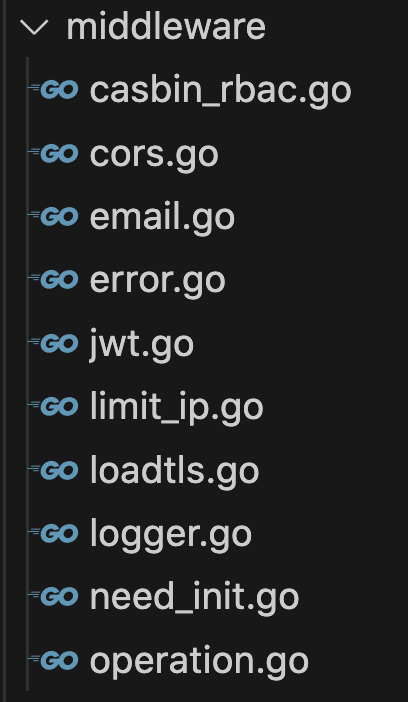
casbin_rbac.go
1 | package middleware |
cors.go
1 | package middleware |
email.go
1 | package middleware |
error.go
1 | package middleware |
jwt.go
1 | package middleware |
limit_ip.go
1 | package middleware |
loadtls.go
1 | package middleware |
logger.go
1 | package middleware |
need_init.go
1 | package middleware |
operation.go
1 | package middleware |