思考并回答以下问题:
本次实验将介绍Go语言以及Gin框架的测试。测试是开发网络应用的重要工作,有助于我们编写健壮稳定的系统和应用。通过本次实验,我们将了解如何编写Go语言自动化测试用例,以及如何编写并集成Gin框架的测试用例。
知识点
- 单元测试
单元测试
下面我们来实战如何在Gin框架中编写单元测试代码。
安装gin后,创建main_test.go,输入以下内容。1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42package main
import (
"net/http"
"net/http/httptest"
"testing"
"github.com/gin-gonic/gin"
)
func TestGetHelloHandler(t *testing.T) {
// 创建一个Gin的Router实例
router := gin.Default()
// 定义GET请求的路由处理函数
router.GET("/hello", func(c *gin.Context) {
c.JSON(http.StatusOK, gin.H{"message": "Hello, World!"})
})
// 创建一个HTTP请求的记录器
recorder := httptest.NewRecorder()
// 创建一个GET请求的HTTP请求
req, err := http.NewRequest("GET", "/hello", nil)
if err != nil {
t.Fatal(err)
}
// 使用Gin的Router处理HTTP请求
router.ServeHTTP(recorder, req)
// 检查HTTP响应的状态码是否为200
if recorder.Code != http.StatusOK {
t.Errorf("Expected status code %d, but got %d", http.StatusOK, recorder.Code)
}
// 检查HTTP响应的消息体是否包含预期的内容
expected := `{"message":"Hello, World!"}`
if recorder.Body.String() != expected {
t.Errorf("Expected response body %s, but got %s", expected, recorder.Body.String())
}
}
在上面的单元测试用例中,我们创建了一个/hello的GET请求控制器,并返回{"message":"Hello,World!"}
的JSON数据。然后我们通过httptest.NewRecorder
实例recorder来进行HTTP请求,然后判断返回信息是否正确。
创建好文件后,我们打开终端,输入go test
,可以得到如下结果。
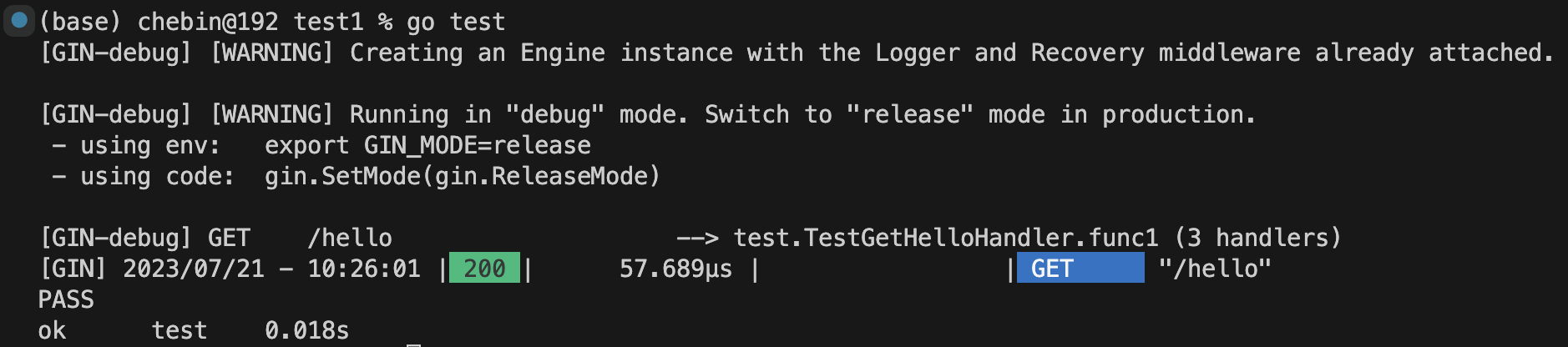